Create event-driven applications with Cloudflare Queues and Dapr
In this post, you'll learn how to build a cloud to edge event-driven application with Dapr and Cloudflare Queues.

In this post, you'll learn how to build a cloud to edge event-driven application with Dapr and Cloudflare. You'll learn how to create:
- A Cloudflare queue.
- A consumer Cloudflare worker (in TypeScript) that reads messages from the queue.
- A producer Dapr app (in TypeScript) that uses the Cloudflare Queue binding to publish messages to the queue.
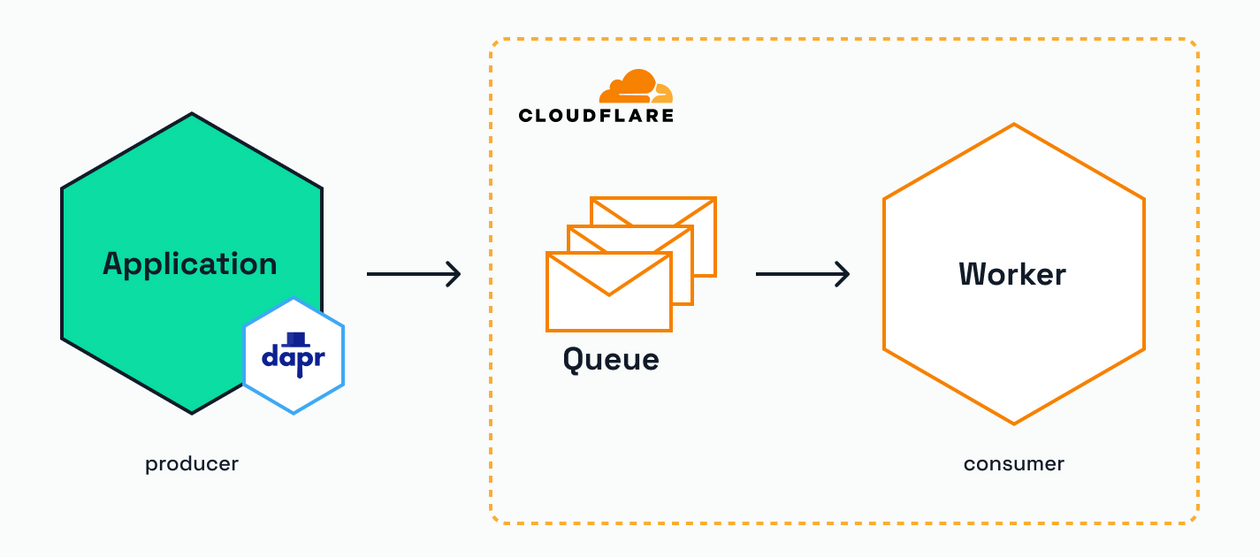
Event-driven applications FTW 🚀
Event-driven applications are becoming increasingly popular. The decoupling of event producers and event consumers makes applications more resilient and flexible, since they allow independent implementation and scaling of the applications.
Cloudflare recently announced Queues, allowing developers to send and receive messages with guaranteed delivery and integrating with Cloudflare Workers, a fast edge computing platform.
Dapr, the open-source distributed application runtime, is often used in event-driven applications. Dapr provides a set of standardized API building blocks that simplify microservice development. By using the Bindings building block, developers can use input, and output bindings, and either trigger their apps or invoke other resources without having to learn resource-specific SDKs for these resources. With Dapr release 1.10, a new binding is provided that allows developers to publish messages to Cloudflare Queues. Because of the common set of APIs that Dapr offers, developers from any background can use the binding to publish messages to Cloudflare Queues without needing to know the Cloudflare SDKs or adding that dependency to their codebase.
Let's start building an event-driven app and see the binding in action.
Prerequisites
The following is required to run this sample:
- Clone this repository to your local machine.
- Install Dapr CLI
- Use the Dapr CLI to install the Dapr runtime locally: dapr init
- Install Node.js
- Install Cloudflare Wrangler
- Ensure you're on a Cloudflare paid plan, since that is required to use Cloudflare queues.
- Enable Queues in the Cloudflare dashboard.
- Dashboard > Workers > Queues
- Enable Queues Beta
- You should see a confirmation in the dashboard that queues are enabled.
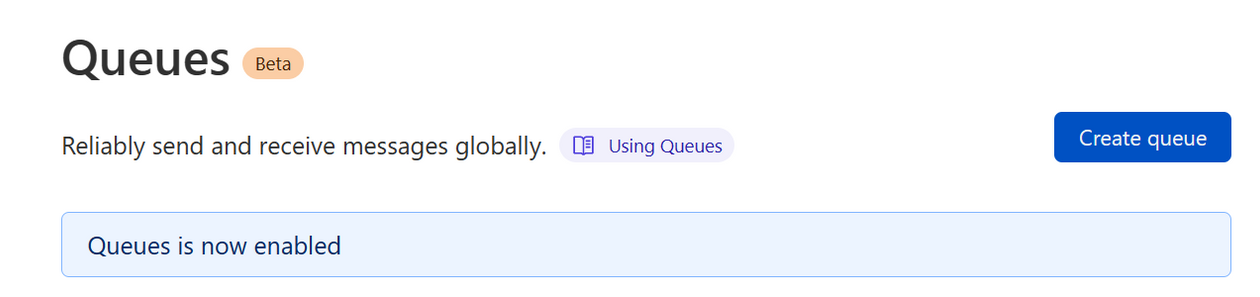
Create the applications
The solution consists of three parts:
- A Cloudflare queue
- A consumer Cloudflare worker that reads messages from the queue.
- A producer Dapr app that will publish messages to the queue.
1. Create a Cloudflare queue
1. Open a terminal and use the wrangler CLI to login to Cloudflare:
<inline-h>wrangler login<inline-h>
Follow the instructions in the browser to login to Cloudflare.
The response in the terminal should end with:
<inline-h>Successfully logged in<inline-h>.
2.Create the Cloudflare queue using the wrangler CLI:
<inline-h>wrangler queues create dapr-messages<inline-h>
The response in the terminal should end with:
<inline-h>Created queue dapr-messages<inline-h>.
2. Create a consumer Cloudflare worker
You can either create a new consumer worker by following steps 1-3, or use the existing consumer worker in this repository and continue from step 4.
1. In the root folder, create a worker to consume messages:
<inline-h>wrangler init consumer<inline-h>
- Create package.json: <inline-h>Y<inline-h>
- Use TypeScript: <inline-h>Y<inline-h>
- Create worker: <inline-h>Fetch handler<inline-h>
- Write tests: <inline-h>N<inline-h>
A new folder named consumer will be created which contains the worker.
2. Update the consumer/src/index.ts file to:
3. Add the following lines to the consumer/wrangler.toml file:
4. Ensure that you're in the consumer folder and install the dependencies:
<inline-h>cd consumer<inline-h>
npm install
5. Publish the consumer worker:
<inline-h>wrangler publish<inline-h>
The response in the terminal should end with:
6. Start a tail to read the log of the consumer worker:
<inline-h>wrangler tail<inline-h>
3. Configure the producer Dapr app
The Cloudflare Dapr binding uses a Cloudflare worker to publish messages, since only Cloudflare workers can access the queue.
There are two options for this worker:
- Dapr provisions the worker.
- You use a pre-provisioned Cloudflare worker.
This sample uses option 1. Read the Cloudflare Queues binding spec and choose Manually provision the Worker script if you want to go for option 2.
Create a binding file
- Rename the<inline-h> producer/resources/binding.yaml.template<inline-h> to <inline-h>producer/resources/binding.yaml<inline-h>.
- Open the <inline-h>binding.yaml<inline-h> file and inspect its content.
The <inline-h>metadata.name<inline-h>, <inline-h>spec.metadata.queueName<inline-h> and <inline-h>spec.metadata.workerName<inline-h> values have already been set. Ensure that the <inline-h>queueName<inline-h> matches the queue setting in the consumer worker <inline-h>wrangler.toml<inline-h> file.
Values for <inline-h>spec.metadata.key<inline-h>, <inline-h>spec.metadata.cfAccountID<inline-h>, and <inline-h>spec.metadata.cfAPIToken<inline-h> still need to be provided.
3. Follow these instructions in the Dapr docs to set the value for spec.metadata.key.
4. The Cloudflare account ID should go in the <inline-h>spec.metadata.cfAccountID<inline-h> field. You can find the account ID in the Cloudflare dashboard URL: <inline-h>https://dash.cloudflare.com/<ACCOUNT_ID>/workers/overview<inline-h>.
5. A Cloudflare API token should go in the <inline-h>spec.metadata.cfAPIToken<inline-h> field. It can be generated as follows:
- In the Cloudflare dashboard, go to the Workers page.
- Click the API tokens link
- Click the Create token button
- Click the Use template button for Edit Cloudflare Workers
- Update the permissions to only contain:
Account | Worker Scripts | Edit - Update the Account Resources to only contain:
Include | <YOUR ACCOUNT> - Set a time to live (TTL) for the token, the shorter, the better, if you're just testing.
Now the binding file is complete. The file is gitignored, so the secrets won't be committed to the repository.
Inspect the Node app
Let's have a look at the Dapr app that will send the messages to the Cloudflare queue.
- Inspect the producer/index.ts\ file.
Note that the `bindingName` is set to `cloudflare-queues` and matches the value of the `metadata.name` property in the `binding.yaml`. The `bindingOperation` is set to `publish` (`create` could be used as an alias).
Run the producer app
1. Open a new terminal window and navigate to the `producer` folder.
2. Run npm install to install the dependencies:
3. Run the following command to start the producer app:
4. The terminal that logs the tail of the consumer app should show a log statement for each of the ten messages sent:

Congratulations! You've now sent messages from a Dapr app to a Cloudflare Queue (& Worker) via the Cloudflare Queues binding! 🎉 Hungry for more Dapr bindings? Have a look at the long list of supported bindings.
Any questions or comments about this blog post or the code? Join the Dapr discord and post a message in the `#components-contrib` channel. Have you made something with Cloudflare and Dapr? Post a message in the `#show-and-tell` channel, we love to see your creations!
Diagrid Newsletter
Subscribe today for exclusive Dapr insights